- Is positioned above the widget just over the top left corner
- It only appears when the user has 'Alt' pressed
- Clicking it causes the widget to close (with a nice swoosh animation)
Well, the animation will have to wait, but the button is easy to knock up in Swing.
- The button will extend AbstractButton and draw it's own close icon of a white cross inside a black disc.
- The button will listen for mouse clicks inside the disc area, and call System.exit(0) to close the widget.
- The widget frame has a JLayeredPane allowing arbitrary placement of the button above the main panel
- Add input/action entries on the widget frame to respond to the alt key and call button.setVisible(true/false).
In the main widget frame class add the button to a high layer, and set up the 'Alt' input actions:
Gives this beauty...
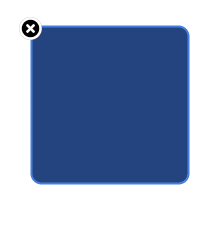
Update: Pressing the 'Alt' key is pretty unintuitive for users to close the widgets. Dashboard has a widget manager which offers an alternate more intuitive method to close them. As the Java widgets are trying to avoid the need for a widget engine, I'll just make the close button fade in and out as the mouse moves in or out of the widget - just like the info button in the next part...